Hey David,
I've implemented a simple report with an ArrayDataSource
both as a data source in the report settings and as a generated array in the OnRunEnd()
function to replicate the behavior of my main report as closely as possible.
- The name of the Excel Template file was
SimpleReport.excel.php
- The export command was:
$reportClass->run()->exportToExcel($currentReport)->toBrowser($currentReport . '.xlsx');
(The $reportClass
and $currentReport
variables are passed as GET parameters to my site)
Also here are the sources of my simple report:
SimpleReport.php
<?php
use koolreport\datasources\ArrayDataSource;
require_once 'vendor/autoload.php';
class SimpleReport extends koolreport\KoolReport {
use \koolreport\export\Exportable;
use \koolreport\excel\ExcelExportable;
protected function settings() {
return array(
"dataSources"=>array(
"array_example_datasource"=>array(
"class"=>'\koolreport\datasources\ArrayDataSource',
"dataFormat"=>"associate",
"data"=>array(
array("customerName"=>"Johny Deep","dollar_sales"=>100),
array("customerName"=>"Angelina Jolie","dollar_sales"=>200),
array("customerName"=>"Brad Pitt","dollar_sales"=>200),
array("customerName"=>"Nicole Kidman","dollar_sales"=>100),
)
),
)
);
}
protected function setup() {
$this->src('array_example_datasource')
->pipe($this->dataStore('sales_by_associate'));
}
/**
* Emitted after report is run
*
* @return void
* @throws Exception
*/
protected function OnRunEnd()
{
$dataSet2 = array(
array("Name" => "Microsoft", "Products" => "Office, Windows, Azure"),
array("Name" => "Samsung", "Products" => "Phones, Fridges, Laptops"),
array("Name" => "Sony", "Products" => "Cameras, Phones, Radios")
);
$backupReportCommentTable = new ArrayDataSource();
$backupReportCommentTable->load($dataSet2)
->start();
$backupReportCommentTable->pipe($this->dataStore('dataSet2'));
}
}
SimpleReport.view.php
<?php
use \koolreport\widgets\koolphp\Table;
?>
<div class="report-content">
<div class="text-center">
<h1>Sales By Associate</h1>
</div>
<?php
Table::create(array(
"dataStore"=>$this->dataStore('sales_by_associate')
));
?>
<?php
Table::create(array(
"dataStore"=>$this->dataStore('dataSet2')
));
?>
</div>
SimpleReport.excel.php
<?php
use \koolreport\excel\Table;
$sheet1 = "Simple report";
?>
<meta charset="UTF-8">
<meta name="description" content="Very simple report for testing">
<meta name="keywords" content="Excel,HTML,CSS,XML,JavaScript">
<meta name="creator" content="Koolreport">
<meta name="subject" content="">
<meta name="title" content="Simple report">
<meta name="category" content="Report">
<meta name="company" content="Advanced Applications GmbH">
<div sheet-name="<?php echo $sheet1; ?>">
<div>Simple report</div>
<div>
<?php
Table::create(array(
"dataSource" => $this->dataStore('array_example_datasource')
));
?>
</div>
</div>
<div sheet-name="Companies">
<div>Simple report</div>
<div>
<?php
Table::create(array(
"dataSource" => $this->dataStore('dataSet2')
));
?>
</div>
</div>
The behavior is the same as with my main report; the HTML renders fine but the Excel is empty except for the titles. Here is a preview of the rendered HTML:
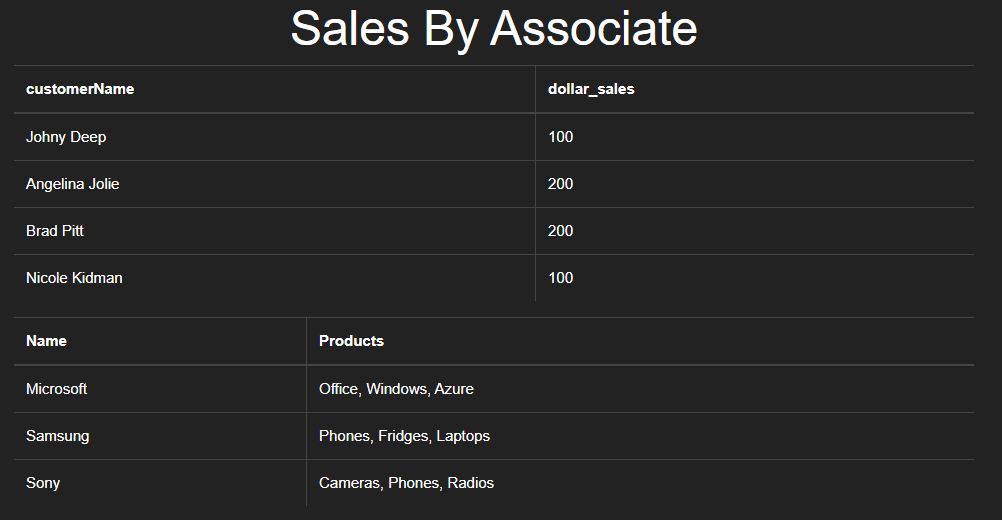