here is code for my Order Summary from where I want to use Export feature ( initially only PDF, will try Excel after success ).
\App\Controllers\Ordersummary.php
<?php
namespace App\Controllers;
require APPPATH . "Reports/OrderSummary/OrderSummary.php";
class Ordersummary extends BaseController
{
public function index(){
$report = new \App\Reports\OrderSummary();
$report->run();
$data['report'] = $report;
echo view('reports/OrderSummary', $data);
$this->modulename = 'Order Summary';
}
public function RedirectTo() {
return redirect()->to('/');
}
\App\Reports\OrderSummary\OrderSummary.php
<?php
namespace App\Reports;
require_once ROOTPATH . "load.koolreport.php";
use \koolreport\KoolReport;
use \koolreport\processes\Sort;
class OrderSummary extends \koolreport\KoolReport
{
//use \koolreport\bootstrap4\Theme;
use \koolreport\amazing\Theme;
use \koolreport\codeigniter\Friendship;
use \koolreport\inputs\Bindable;
use \koolreport\inputs\POSTBinding;
protected function defaultParamValues()
{
return array(
"dateRange"=>array(
"2022-12-01",
"2022-12-10",
),
);
}
protected function bindParamsToInputs()
{
return array(
"dateRange"=>"dateRange",
);
}
function setup()
{
$this->src("default")
->query("SELECT DATE(order_date) AS order_date, no_of_orders, rider_charges, order_total FROM order_summary WHERE
order_date >= :start
AND
order_date <= :end")
->params(array(
":start"=>$this->params["dateRange"][0],
":end"=>$this->params["dateRange"][1],
))
->pipe(Sort::process([
"order_date"=>"desc"
]))
->pipe($this->dataStore("result"));
}
}
\App\Reports\OrderSummary\OrderSummary.view.php
<?php
use \koolreport\widgets\koolphp\Table;
use \koolreport\inputs\DateRangePicker;
use \koolreport\inputs\Select2;
?>
<style>
.amazing {
width: 40%;
}
.select2 {
width: 100% !important;
}
.sidebar {
background: #ffffff !important;
}
</style>
<div class='report-content'>
<div class="text-center">
<h2>Orders Summary</h2>
<a href="export.php" class="btn btn-primary">Download PDF</a>
</div>
<form method="post">
<div class="row">
<div class="col-md-8 offset-md-2">
<div class="form-group" style="display: flex; flex-direction: row; flex-wrap: nowrap; align-content: center; justify-content: center; align-items: center; margin-bottom: 1rem;">
<?php
DateRangePicker::create(array(
"name"=>"dateRange",
"format"=>"YYYY-MM-DD"
))
?>
<div class="form-group text-center" style="margin-left: 1rem;margin-bottom: 0rem;">
<button class="btn btn-success"><i class="glyphicon glyphicon-refresh"></i> Load</button>
</div>
</div>
</div>
</form>
</div>
</div>
<script>
function Backonclick() {
window.location.href = '<?php echo base_url(); ?>/ordersummary/back';
}
</script>
<?php
if($this->dataStore("result")->countData()>0)
{
Table::create(array(
"dataStore"=>$this->dataStore("result"),
"cssClass"=>array(
"table"=>"table table-bordered"
),
"showFooter"=>true,
"options"=>array(
"paging"=>true,
),
"columns"=>array(
"order_date"=>array(
"cssStyle"=>"text-align:center",
"label"=>"Order Date",
"footerText"=>"<b>Total</b>"
),
"no_of_orders"=>array(
"cssStyle"=>"text-align:right",
"label"=>"No. of Orders",
"type"=>"number",
"footer"=>"sum",
"footerText"=>"<b>@value</b>"
),
"rider_charges"=>array(
"cssStyle"=>"text-align:right",
"label"=>"DC",
"type"=>"number",
"footer"=>"sum",
"footerText"=>"<b>@value</b>"
),
"order_total"=>array(
"cssStyle"=>"text-align:right",
"label"=>"GMV",
"type"=>"number",
"footer"=>"sum",
"footerText"=>"<b>@value</b>"
),
),
"paging"=>array(
"pageSize"=>10,
),
));
}
else
{
?>
<div class="alert alert-warning">
<i class="glyphicon glyphicon-info-sign"></i> Sorry, we found no orders.
</div>
<?php
}
?>
</div>
\App\Reports\OrderSummary\export.php
<?php
require_once "OrderSummary.php";
$report = new OrderSummary;
$report->run()
->export('OrderSummaryPdf')
->settings([
// "useLocalTempFolder" => true,
])
->pdf(array(
"format"=>"A4",
"orientation"=>"portrait",
//"zoom"=>2
))
->toBrowser("order_summary.pdf");
\App\Reports\OrderSummary\OrderSummaryPdf.view.php
<?php
use \koolreport\widgets\koolphp\Table;
use \koolreport\widgets\google\ColumnChart;
?>
<html>
<body style="margin:0.5in 1in 0.5in 1in">
<link rel="stylesheet" href="../assets/bs3/bootstrap.min.css" />
<link rel="stylesheet" href="../assets/bs3/bootstrap-theme.min.css" />
<div class="page-header" style="text-align:right"><i>Order Summary Report</i></div>
<div class="page-footer" style="text-align:right">{pageNum}</div>
<div class="text-center">
<h1>Order Summary Report</h1>
<h4>This report show Order's Summary</h4>
</div>
<hr/>
<?php
Table::create(array(
"dataStore"=>$this->dataStore('result'),
"columns"=>array(
"order_date"=>array(
"cssStyle"=>"text-align:center",
"label"=>"Order Date",
"footerText"=>"<b>Total</b>"
),
"no_of_orders"=>array(
"cssStyle"=>"text-align:right",
"label"=>"No. of Orders",
"type"=>"number",
"footer"=>"sum",
"footerText"=>"<b>@value</b>"
),
"rider_charges"=>array(
"cssStyle"=>"text-align:right",
"label"=>"DC",
"type"=>"number",
"footer"=>"sum",
"footerText"=>"<b>@value</b>"
),
"order_total"=>array(
"cssStyle"=>"text-align:right",
"label"=>"GMV",
"type"=>"number",
"footer"=>"sum",
"footerText"=>"<b>@value</b>"
),
),
"cssClass"=>array(
"table"=>"table table-hover table-bordered"
)
));
?>
</body>
</html>
Screenshot: now what it throws
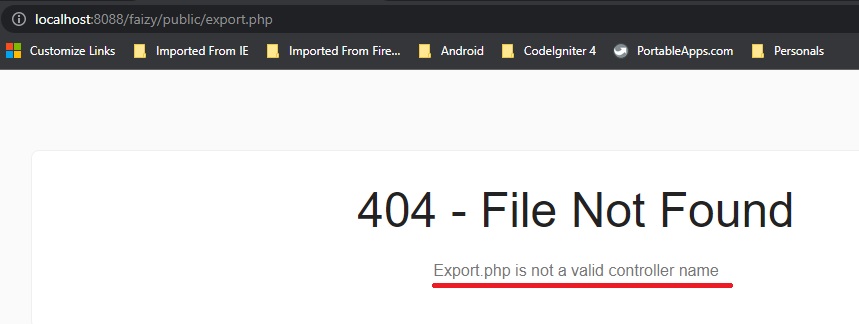
hope these will help to understand.. please help.
regards